What is AWS API Gateway?
Amazon Web Services API Gateway is a managed service for publishing, maintaining, securing, and monitoring APIs at scale. You can leverage the AWS management console to create a front door for your applications to reach business logic, functionality, or data from the back end. These include applications that run on Amazon Elastic Container Service (ECS), Elastic Compute Cloud (EC2), or Elastic Beanstalk, as well as web applications and code running on Lambda.
API Gateway handles all tasks required to accept and process large volumes of simultaneous or concurrent API calls – it can support hundreds of thousands of calls. These tasks include authorization, access control, traffic management, API version management, and monitoring.
Read more about API gateways in a cloud native world
Amazon API Gateway use cases
Creating REST APIs
API Gateway allows you to build REST APIs consisting of resources and methods. A method reflects an API request submitted by a user and the corresponding response, while a resource is a piece of logic that applications can access via a resource path.
The application does not need to know the location of the data requested on the back end. An API Gateway REST API encapsulates the front end using method requests and responses – the API uses integration requests and responses to communicate with the back end.
Creating HTTP APIs
An HTTP API allows you to build a RESTful (stateless) API at a lower cost and latency than a REST API. HTTP APIs are useful for sending requests to a Lambda function or publicly routable HTTP endpoints.
An API Gateway HTTP API supports authorization with OpenID Connect (OIC) and OAuth 2.0. It supports automatic deployments and cross-origin resource sharing (CORS).
Creating WebSocket APIs
In WebSocket (stateful) APIs, both the server and client can communicate with each other anytime. A back end server can easily forward data to a connected device or user without implementing a complex polling mechanism.
A WebSocket API is useful for building secure applications with real-time messaging. You don't have to manage or provision servers to handle connections and massive data exchanges.
WEBINAR: Is it time to replace your Amazon API Gateway with Gloo Edge?
AWS API Gateway architecture and features
Here is a diagram showing the architecture of AWS API Gateway.
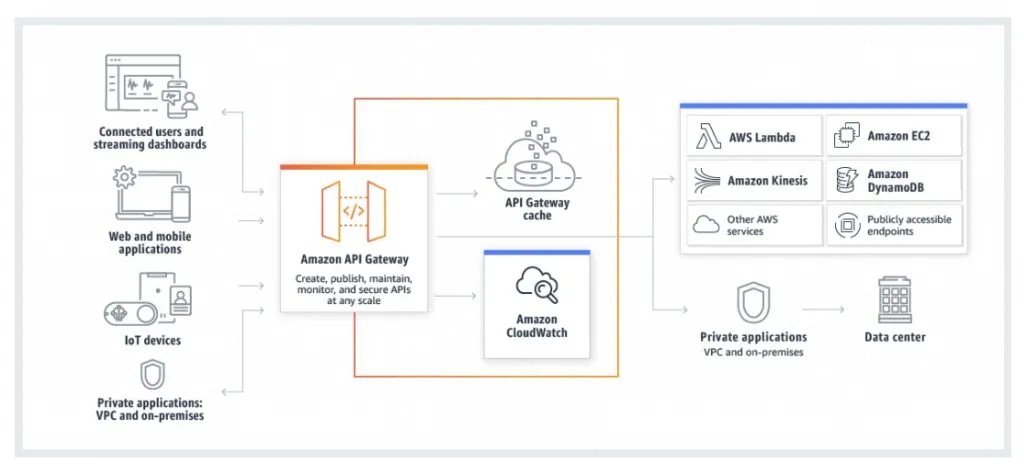
AWS API Gateway supports stateless (e.g., HTTP, REST) and stateful (e.g., WebSocket) APIs. The service provides powerful authentication with AWS IAM policies, user pools from Amazon Cognito, and Lambda functions like authorizers.
API Gateway also offers a developer portal to publish APIs. It integrates with AWS X-RAY to help you understand and triage performance issues and latencies and with AWS WAF to protect APIs from common web-based exploits. You can use CloudTrail to monitor and log changes to your APIs and their usage. Finally, API Gateway supports canary deployments to roll out changes safely.
How does Amazon API Gateway work?
Defining an API
To create an API using Amazon API Gateway, developers need to define four things – a name, a function (HTTP), the API's integration with services, and how transfers and requests should be handled.
Once these basic parameters are defined, developers use any software development kit (SDK) to integrate with the software calling the API. The SDK could be a custom kit for web or mobile applications.
Payloads
Amazon API Gateway will accept any payload sent over HTTP – including JSON (JavaScript Object Notation) and XML (Extensible Markup Language). AWS users can monitor API calls via the API Gateway metrics dashboard. You can also get error, debug, and access logs from CloudWatch.
Handling API traffic
Amazon API Gateway offers two ways to handle API traffic:
- Throttling—restricts the number of API calls allowed per day or hour. Throttling helps maintain application call performance during unexpected spikes in API calls.
- Caching—many API calls process the same information to return the same results. Instead of redoing all the processing necessary to produce a specific result, the cache provides a generic API response. Caching can reduce the number of calls, improving the performance of the calling application.
Securing APIs
API Gateway provides API access control using access keys. Keys grant access to APIs through Amazon Cognito and AWS Identity and Access Management (IAM). Alternative security options include:
- Requiring an AWS Signature (version 4)
- Creating an access key for each API call
- Passing OAuth tokens
Versioning
The AWS API Gateway service allows developers using AWS to use multiple versions of the API simultaneously. This makes it possible to build and deploy new APIs while existing applications use an older version.
AWS API Gateway pricing
To understand how API Gateway billing works, you must first understand the available types of API Gateways: Websocket, REST, and HTTP.
- WebSocket APIs address the problem of how to actively send data to the client. They solve this by sustaining a connection to the client so that it can send data when needed.
- REST APIs is the basic type supported by API Gateway. This is a feature-rich solution for creating serverless REST APIs. The disadvantages are that it is more expensive than the HTTP option, has higher latency, and cannot actively send events to the client.
- HTTP APIs are a relatively new option. They have fewer features, but are lower-cost and lower-latency than REST APIs. In most cases, this will be your preferred option, unless you need specific functionality provided by a REST API.
REST and HTTP APIs are both billed based on the number of received requests. By contrast, because the WebSocket API must maintain several client connections, you are charged for how long a connection remains open and for the number of messages sent and received.
API Gateway works like any other serverless service provided by AWS, on a pay-as-you-go basis. Therefore, you don't pay for idle resources. The amount charged is directly related to how much you actually use the resource.
If you have recently set up an AWS account, you can use the free tier. The API Gateway free tier includes 1 million HTTP API calls, 1 million REST API calls, 1 million messages, and 750,000 connections per month for up to 12 months.
Amazon API Gateway Lambda tutorial
This tutorial explains how to build an API with a back-end AWS Lambda function. You can operate the API free of charge under the AWS Free Tier (provided you have a new Amazon account).
Step 1: Create a Lambda function
We’ll use a Lambda function for the API backend. Lambda will run the code only when needed and automatically scale depending on actual requests made.
We’ll build a sample API using the default Node.js function from the Lambda console.
To create an AWS Lambda function as a back-end for the API:
- Log in to the AWS Lambda Console.
- Click Create Function.
- Enter a name for your function such as example-function.
- Click Create Function.
The example function returns a 200 response to the client with the text Hello from Lambda!
Step 2: Create an HTTP API
Now we’ll create an HTTP API using AWS API Gateway (this is the service option with limited functionality and lower cost). The HTTP API provides an HTTP endpoint for your Lambda function. API Gateway routes the request to the specified Lambda function and returns its response to the client.
To create an HTTP API:
- Open the Amazon API Gateway Console and sign in.
- Click Create API and select Build for HTTP API.
- Select Integrations > Add Integration > Lambda.
- Under Lambda function, type the name of the function defined in the previous section—
example-function
. - Select a name for the API, we’ll use
example-http-api
. - Click Next, review the routing details and steps, then click Create.
Now that you have created an HTTP API with your Lambda integration, you are ready to receive requests from clients.
Step 3: Test your API
Test the API to see if it works. We’ll use a web browser to call the API.
To test the new API:
- Open the API Gateway Console.
- Select your API—
example-http-api
. - Copy the API call URL, shown in the screenshot below.
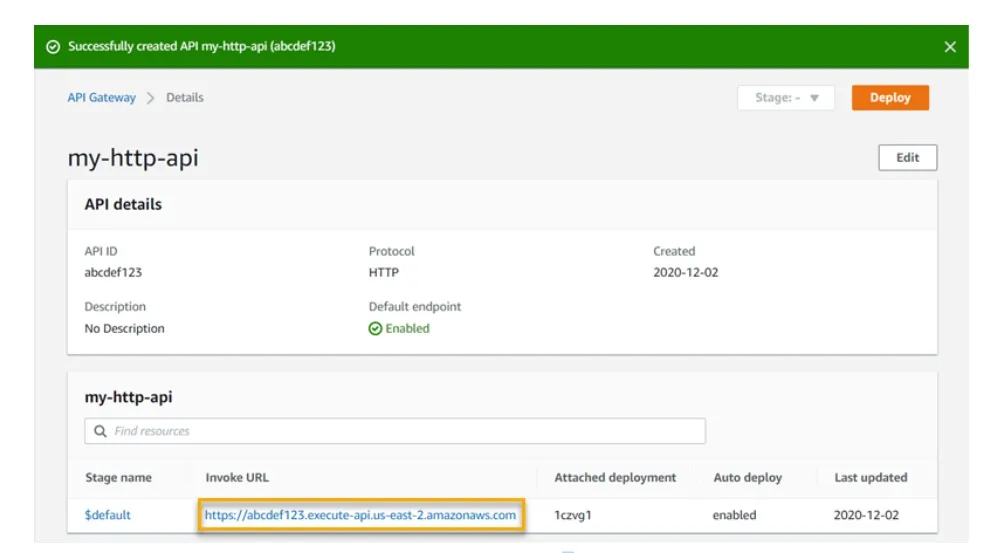
- Open a web browser and paste the call URL. To invoke the Lambda function, append the name of the function to the invocation URL. It should look something like this:https://abcdef123.execute-api.us-east-2.amazonaws.com/example-function
- To see what is happening, open Chrome developer tools and switch to the networking tab. The browser will now send a GET request to the API.
- In the browser, you should see the text “Hello from Lambda!”. This confirms the API is working.
Best practices for securing Amazon API Gateway
API Gateway provides many security features that can help you define and implement your own security policies. The following best practices are general guidelines and do not represent a complete security solution – they may or may not be appropriate for your environment:
- Implement least-privileged access—use IAM policies to enforce least-privilege access to create, read, update, or delete API Gateway APIs. API Gateway provides several options for controlling access to the APIs you create.
- Implement logging—log requests to the API using CloudWatch Logs or Amazon Kinesis Data Firehose.
- Use Amazon CloudWatch alarms—monitor a single metric related to your API over a specified time period using CloudWatch alarms. When a metric exceeds a specified threshold, a notification is sent to an Amazon Simple Notification Service (SNS) topic or an AWS Auto Scaling policy.
- Enable AWS CloudTrail—CloudTrail provides logging of actions taken by users, roles, or AWS services in API Gateway. You can use this information to identify a request to API Gateway, the requested IP address, who made the request, when the request was made, and other details. This can be useful for incident investigation.
- Enable AWS configuration—AWS Config provides a detailed view of the configuration of AWS resources in your account. It shows resource dependencies and a full history of configuration changes. You can use AWS Config to define rules that evaluate the configuration for compliance purposes. AWS Config can also use Amazon SNS topics to notify users when a resource violates rules and is marked as non-compliant.
- Use AWS Web Application Firewall (WAF)—AWS WAF is a service that protects web applications and APIs from cyber attacks. It can help protect API Gateway APIs from common web-based attacks, such as SQL injection and cross-site scripting (XSS).
Using Solo Gloo Gateway as an AWS API Gateway alternative
For many companies, Solo Gloo Gateway is a preferred API Gateway alternative to AWS API-GW, due to its performance, scalability, multi-cloud functionality, Kubernetes-native integration, and overall cost savings (often up to 40%). In addition to robust API-GW security, authorization and authentication, Solo Gloo Gateway also integrates seamlessly with AWS Lambda to enable serverless functionality.